Building a ChatBot using Python + Cursor + Claude for complete beginners
Draft article in progress - feedback welcome.
This is AI Augmented content - I make a list of topics and with the help of perplexity.ai I turned it into the following tutorial.
Introduction
A friend was asking, “where do I get started with AI Engineering skills?” So here it is a lightly edited guide to Building a ChatBot using Python Cursor and Claude.
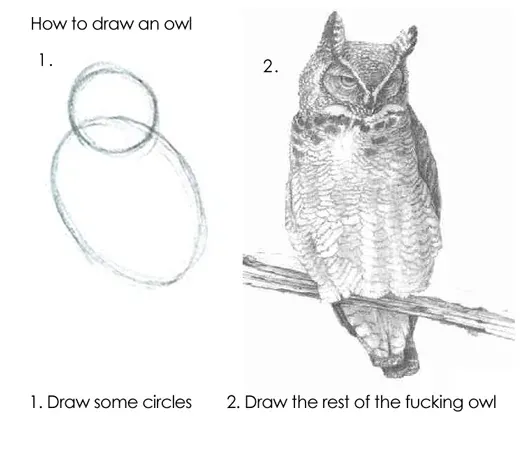
This is based on what I would take someone thought live, but not in much detail, so lots of self-learning will be required if you want to do this on your own.
In this tutorial we suggest you:
- Set up the necessary tools and APIs
- Write and run your first Python program
- Interact with Claude AI using Python
- Create a web interface for your chatbot
- Debug and improve your code with AI assistance
- Deploy your finished chatbot to the web
By the end of this guide, you'll have hands-on experience with AI programming, web development, and deployment processes. Let's get started on your journey to creating your very own AI chatbot!
1. Get an API key from Anthropic
To access Claude AI, you'll need an API key from Anthropic:
- Go to https://console.anthropic.com
- Sign up for an account if you don't have one
- Navigate to the API section
- Generate a new API key
For detailed instructions, check out the “ask the docs” tool: https://docs.anthropic.com/en/home
2. Download Cursor AI
Cursor AI is an AI-powered code editor that will help you write and debug code:
- Visit https://www.cursor.so/
- Click on the "Download" button
- Choose the appropriate version for your operating system (Windows, macOS, or Linux)
- Install the application
3. Configure Cursor to use your API key
To use your Anthropic API key in Cursor:
- Open Cursor AI
- Go to Settings (usually accessible via a gear icon or in the menu)
- Look for a section related to "API Keys" or "Anthropic"
- Enter your API key in the designated field
- Save the settings
For more detailed instructions, refer to Cursor's documentation: https://docs.cursor.com/advanced/api-keys
4. Install Python
For Windows: Follow the guide a https://learn.microsoft.com/en-us/windows/python/web-frameworks
For macOS: Follow the guide at https://www.linkedin.com/pulse/beginners-guide-installing-python-mac-rany-elhousieny-phdᴬᴮᴰ/
5. Write and run "Hello World"
- Open Cursor AI
- Create a new file named
hello_world.py
- Type the following code:
print("Hello, World!")
- Save the file
- Run the program using the built-in terminal in Cursor or by right-clicking and selecting "Run Python File"
python hello_world.py
You should see "Hello, World!" printed in the terminal output.
6. Creating a requirements.txt file
- Create a new file named
requirements.txt
- Add the following line to the file:
anthropic
- Save the file
7. Creating a virtual environment in Cursor
- Open the terminal in Cursor
- Navigate to your project directory
- Create a virtual environment:
python -m venv .venv
- Activate the virtual environment:
- On Windows:
.venv\\Scripts\\activate
- On macOS/Linux:
source .venv/bin/activate
- On Windows:
- Install the requirements:
pip install -r requirements.txt
8. Write a Python program using Claude
Use Cursor's AI capabilities to generate a program that interacts with Claude:
Create a new file named
claude_chat.py
Use Cursor's AI to generate the code by using Command-K and typing an instruction like:
Write a program that sends a question to Claude using the anthropic library and prints the response
Cursor should generate code similar to this:
import anthropic
client = anthropic.Anthropic(
# defaults to os.environ.get("ANTHROPIC_API_KEY")
api_key="my_api_key",
)
message = client.messages.create(
model="claude-3-haiku-20240307",
max_tokens=4096,
temperature=0,
messages=[
{
"role": "user",
"content": [
{
"type": "text",
"text": "hello"
}
]
}
]
)
print(message.content)
- Replace "YOUR_API_KEY" with your actual Anthropic API key (or better yet set the environment variable so the key is not in your source code)
- Run the program
9. Providing links to documentation in Cursor chat
If cursor generates out of date or incorrect code try giving it up-to-date information:
- In the Cursor chat, type
@web
followed by your search query - Cursor will search the web and provide relevant links
You can also paste in a link to documentation (eg the link to https://docs.anthropic.com/en/api/getting-started) in the chat and ask Cursor to index that documentation, and you can re-use it in future prompts.
10. Debugging with Cursor
If you encounter an error:
- Copy the error message
- Paste it into the Cursor chat (or use the Ctrl-L “add to chat” feature)
- Ask Cursor to explain the error and suggest a fix
- Apply the suggested fix to your code
11. Adding a user input loop
To add a user input loop using inline AI editing:
Place your cursor where you want to add the loop
Type a comment like:
# Add a user input loop to continuously chat with Claude
Cursor should suggest code to implement the loop
If you are on the free plan you can also press Ctrl-K to bring up the inline code generation, and give the instruction there
You can stop here if you want! You have a working chatbot, but it’s on the command line, and you might want something more flashy on the web. If so:
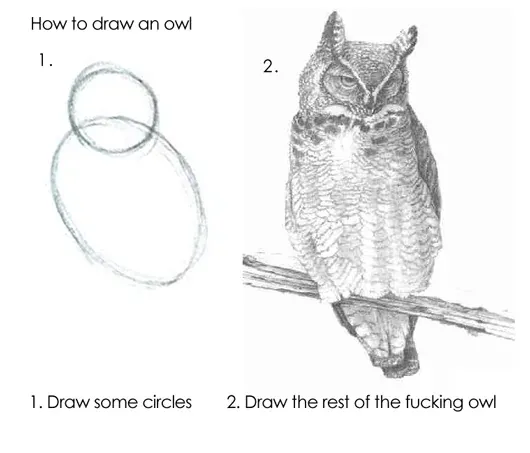
12. Building a web interface
To create a web interface using Flask:
- Ask Cursor: "How can I build a web interface for this program using Flask?"
- Follow Cursor's suggestions to create a basic Flask app
- Add Flask to your
requirements.txt
file and reinstall requirements
13. Building the Flask app
- Ask Cursor to generate the Flask app code
- Follow the instructions to add necessary libraries and create required files
- Run the Flask app and test it in your browser
14. Iterating and debugging the web app
- Test the web app thoroughly
- If you encounter issues, describe them to Cursor and ask for help
- Apply Cursor's suggestions and continue iterating
15. Using Claude Artifacts and v0 for UI prototyping
- Visit https://claude.ai or https://www.v0.dev/
- Use these tools to generate UI mockups or prototypes
- Share the generated designs with Cursor and ask for implementation suggestions
16. Improving the app with generated code
- Give Cursor the generated UI code
- Ask Cursor to suggest improvements to your Flask app based on these designs
- Implement the suggested changes
17. Deployment options
Ask Cursor: "What are some options for deploying this Flask app?"
Cursor should suggest various platforms like Heroku, AWS, Google Cloud, or Railway.
18. Deploying to Railway
- Visit https://railway.app/
- Sign up for an account
- Follow Railway's documentation to deploy your Flask app
- Ask Cursor for help with any specific deployment steps or issues
Remember to iterate and improve your app based on feedback and testing. Good luck with your AI engineering journey!
Getting more advanced
Where to from here? I find the modern web frontend tech (things like NextJS and React) generally a bit complicated and not ideal for beginners but if you are interested there is an excellent example of a more advanced ChatBot application quickstart provided by Anthropic.